Dec 31, 2020
The number of people who have asked me to share Flutter Resources they can use to learn development has been insane! And since I started my Flutter blog, the number has grown exponentially.
I hate giving half-hearted responses, so I decided to create a massive and organized list of resources to help aspiring developers grow and stay organized. This list contains articles, YouTube videos, courses (non-affiliate), and even GitHub repositories that will help you kick off your Flutter development.
Although this list can be used as a roadmap, I don’t recommend it, reason being that a lot of the topics mentioned are not necessary for professional Flutter development.
For example, you don’t need to learn about every state management solution. Just picking one or two from this list will be good enough to understand the fundamentals.
One more side note. This list is not rigid, meaning that it will be updated from time to time.
Happy Fluttering 🚀
Dart
Dart is the programming language used to build Flutter apps. Before you use Flutter (or even dive into these Flutter resources), you should be at least a little familiar with Dart and programming in general. Here are some Flutter resources that will help you improve your Dart skills!
Introduction
Official Dart Website - Documentation
A Gentler Introduction to Programming - Article
Introduction to Dart - Article
Dart Programming: Full Course - Video
Effective Programming
Effective Dart - Documentation
S.O.L.I.D. The first 5 Principles of Object-Oriented Design in Dart - Article
Design Patterns in Dart - Repository
16 Dart Tips and Tricks Every Flutter Developer Should Know - Article
Asynchronous Programming
Asynchronous Programming: Futures, await, async - Documentation
Asynchronous Programming: Futures - Article
Asynchronous Programming: Streams - Documentation.
Advanced Concepts
Functional Programming in Dart - Slideshow
DartZ - Package
RxDart Explained - Video [Flutter Knowledge Required]
RxDart - Package
Dart (DartLang) Introduction: Advanced Dart Features - Article
Recommended Course
The Complete Dart Language Guide For Beginners and Beyond - Andrea Bizzotto
Welcome To Flutter
Now that you know at least, the fundamentals of Dart programming, you can get started with learning how to develop apps with Flutter 🎉. These Flutter resources cover the basics of creating apps with Flutter.
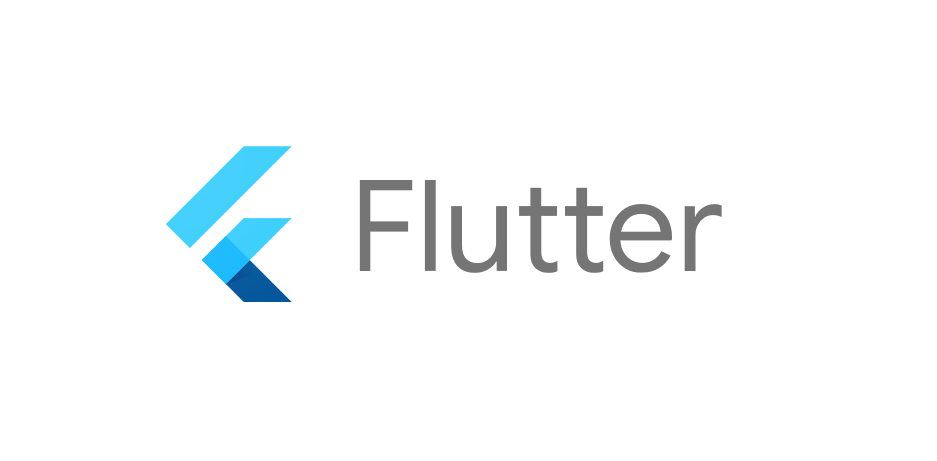
CC - flutter.dev
Official Flutter Website - Documentation
Installing Flutter - Documentation
Setting up an Editor - Documentation
Running the starter template - Documentation
Write your First Flutter App - Codelab
Flutter Tutorial for Beginners - Build iOS and Android Apps with Google’s Flutter and Dart - Video
Flutter Tutorial For Beginners - Free Course
Flutter in 2 hours - Video
Widgets
Introduction to Widgets - Documentation
Widget Catalog - Documentation
Layouts
Layouts in Flutter - Documentation
Flutter layout CheatSheet - Article
Assets
Adding assets and images - Documentation
Navigation and Routing
Navigation cookbook - Documentation
Learning Flutter’s New Navigation and Routing System - Article
Animations
Introduction to Animations - Documentation
Implicit Animations - Codelab
Explicit Animations in Flutter - Video
Json & Serialization
Json & Serialization - Documentation
Flutter: Auto Create Models from JSON | Serializable - Video
Networking
Networking cookbook - Documentation
Http Requests with Flutter - Article
Local Storage
Using SharedPreferences in Flutter - Article
How to use Local Storage in Flutter - Article
Persist data with SQLite - Documentation
Firebase
Note: A lot of Firebase Tutorials are now outdated due to recent package updates. Any potentially outdated Flutter Resources will be marked with an asterisk (*). The most reliable learning resource is the official documentation.
Website - Documentation
Firebase for Flutter - Codelab
Flutter and Firebase App Tutorial* - Free course
Recommended Course
The Complete 2020 Flutter Development Bootcamp with Dart - Dr. Angela Yu
Flutter and Firebase: Build a Complete App for iOS and Android - Andrea Bizzotto
State Management
State management can be very confusing to new Flutter Developers, the reason being that there are so many options.
But you do not need to know how to use every state management solution to be considered a good Flutter Developer.
Just knowing how to manage state using one or two packages and of course, the default state management solution in Flutter should be enough. The following Flutter resources (sorted by package/method) will help you learn the basics of using each solution.
Adding interactivity to your Flutter App - Article
Introduction to State Management - Article
Intro to State Management - Documentation
Pros and Cons of Popular State Management Approaches - Article
Inherited Widget
Inherited Widget - Documentation
Managing Flutter Application State with Inherited Widgets - Article
Provider
Introduction To Provider - Video
Provider Overview for Humans Article
When in doubt, just use Provider - Article
Bloc & Cubit
Website - Documentation
Flutter Bloc and Cubit Tutorial - Video
Flutter Bloc Concepts - Video
Redux
Introduction to Redux in Flutter - Article
MobX
Flutter MobX Crash Course - Video
Working with MobX in Flutter - Article
GetIt + GetIt Mixin
Riverpod
Website - Documentation
Flutter Riverpod Tutorial: Counter App - Article
GetX
The Flutter GetX Ecosystem - Article
GetX In Flutter - Article
Complete GetX State Management - Video
Binder
Application Examples - Repository
Architecture, Structure & Testing
If you’re a fan of writing your UI, application logic, and business logic in a single widget, this section is definitely for you. These Flutter Resources will teach you how to build scalable and maintainable applications using Flutter.
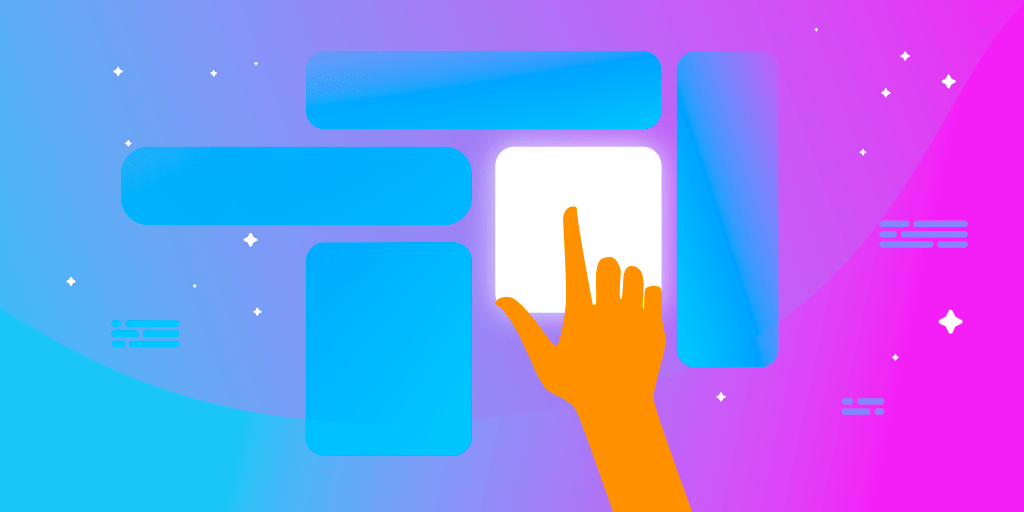
CC - Codemagic.io
Architecture
Flutter Architecture Samples - Repository
Flutter Architecture: Go From setState to Architecture in 20 Mins - Video
Starter Architecture for Flutter & Firebase Apps using Riverpod - Article
Getting Started With the BLoC Pattern - Article
Strategic Domain Driven Design for Improving Flutter Architecture - Talk
Flutter Firebase & DDD Course - Free Course
Flutter TDD Clean Architecture Course - Free Course
Structure
Flutter Scalable Folder & File Structure - Article
Testing
Testing Flutter Apps - Documentation
How to Test Flutter Apps - Codelab
Flutter Testing For Beginners - The Ultimate Guide - Video
Integration Testing - Documentation
Updates on Flutter Testing - Article (105)
Deployment
Now that you’ve built your Flutter app, how do you release it to the stores? The following Flutter Resources cover just that.
Release
Build and Release an Android App - Documentation
Build and Release an iOS App - Documentation
CI/CD
Continuous Delivery with Flutter (fastlane) - Documentation
Contributing to Flutter
Want to make Flutter more fun to work with? Here’s how you can do that!
Packages and Plugins
Developing Packages and Plugins - Documentation
How to Create, Publish and Manage Flutter Packages - Article
How to Write a Flutter Plugin - Codelab
Contributing to The Framework
Contributing to Flutter: Getting Started - Article
Contributing to Flutter - README
Miscellaneous Topics
The next few Flutter Resources cover more specific and advanced topics.
More Widgets
Widget of the Week - Playlist
Deep Dive into Flutter TextField - Article
A Deep Dive into PageView In Flutter - Article
A Deep Dive into DatePicker In Flutter - Article
A Deep Dive into Hero Widgets in Flutter - Article
A Deep Dive into Draggable and Drag Target in Flutter - Article
Flutter Deep Dive: Gestures: Gestures - Article
More Animations
Animation deep dive - Article
Flutter Hooks Tutorial - Video
Use Rive and Flutter for dynamic, interactive, & animated experiences - Talk
Better Development
5 Tools/Packages for Productive Flutter Development - Article
VS Code Extensions Ever Flutter Developer Should Have - Video
Visual Studio Extensions for Fast and Efficient Flutter Development - Article
12 Flutter Tips and Tricks - Video
Flutter Tips (Dart & Flutter Easy Wins) - Article Series
Flutter DevTools - Documentation
Debugging Flutter Apps - Documentation
Debugging (Boring Show) - Video
Performance
Flutter performance best practices - Documentation
Flutter Performance Profiling - Documentation
How to improve the performance of your flutter app - Article
Painting
Painting and Effect Widgets - Documentation
Custom Painting in Flutter - Video
Custom Paint in Flutter - Article
How to Draw And Animate Designs with Flutter CustomPaint Widget - Article
Rendering
Game Development
Building A 2D game in Flutter - Article
Building Games Using Flame - Talk
New: Null Safety
Null Safety in Flutter - Documentation
Migrating to Null Safety - Documentation
More Resources
The following Flutter resources contain a list of websites, creators, tools and repositories to help you improve your Flutter Development.
Official Website: flutter.dev
Official Repository: flutter/flutter
Blogs and Websites
Medium* - medium.com
Reso Coder - resocoder.com
Codemagic - codemagic.io
Self Improving Developer - selfimproving.dev
Code With Andrea - codewithandrea.com
Filled Stacks - filledstacks.com
Super Declarative - superdeclarative.com
Fireship.io - fireship.io
Dash Overflow - dash-overflow.net
Wilson Wilson - wilsonwilson.dev
Iiro.dev - iiro.dev
It’s all widgets - itsallwidgets.com
Jedi Pixels - jedipixels.dev
Youtube Channels
More Resource Collections
Podcasts
Roadmaps
Flutter Roadmap - Repository
Flutter Development Roadmap - Repository
Flutter Development Roadmap 2020 - Repository